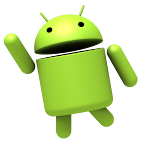
Android uygulamalarında konuma göre (GPS, NETWORK) hava durumu nasıl çekilir.
Başla
Uygulama çalıştırıldığında ilk önce konum bilgisi veren hizmetlerin (GPS vb.) kullanılabilir olup olmadığı kontrol ediliyor. Kullanılabilir ise konum bilgisi hava durumu servisine postalanıyor ve geri dönen JSON veri işlenerek ekrana bastırılıyor. Eğer konum hizmetleri kapalı ise uyarı veriyor ve sizden konum ayar sayfasına gitmenizi istiyor.
Adım 1 :
Gerekli İzinleri Verin
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.gurkan0791.weatherlikeapp" > <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Adım 2 :
Arayüz
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:background="@drawable/background_image" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:id="@+id/textViewWeather" android:background="#ffa72a" android:layout_alignTop="@+id/textView3" android:layout_centerHorizontal="true" /> <ImageView android:layout_width="50dp" android:layout_height="50dp" android:id="@+id/myImageView" android:layout_above="@+id/textViewCountry" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="Country" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginTop="77dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="City" android:id="@+id/textView2" android:layout_marginTop="30dp" android:layout_below="@+id/textView" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="Weather" android:id="@+id/textView3" android:layout_below="@+id/textView2" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginTop="29dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:id="@+id/textViewCountry" android:layout_alignTop="@+id/textView" android:layout_alignLeft="@+id/textViewWeather" android:layout_alignStart="@+id/textViewWeather" android:background="#ffa72a" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:id="@+id/textViewCity" android:layout_alignTop="@+id/textView2" android:layout_alignLeft="@+id/textViewWeather" android:layout_alignStart="@+id/textViewWeather" android:background="#ffa72a" /> </RelativeLayout>
Adım 3 :
Rest Service' den Konum Bilgisine Göre Verileri Çekin. Parse İşlemi Yapın ve Arayüzde Gösterin.
package com.example.gurkan0791.weatherlikeapp; import android.app.ProgressDialog; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.os.AsyncTask; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.widget.ImageView; import android.widget.TextView; import android.widget.Toast; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { //json veri sabitleri private static final String MAIN = "main"; private static final String TEMP = "temp"; private static final String SYS ="sys"; private static final String COUNTRY ="country"; private static final String CITY ="name"; private TextView myTextView; private TextView myTextViewCountry; private TextView getMyTextViewCity; private ImageView myImageView; private static GetWeather getWeather = null; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); myTextView = (TextView) findViewById(R.id.textViewWeather); myTextViewCountry = (TextView) findViewById(R.id.textViewCountry); getMyTextViewCity = (TextView) findViewById(R.id.textViewCity); myImageView = (ImageView) findViewById(R.id.myImageView); //async metodu çalıştır getWeather = new GetWeather(); getWeather.execute(); } @Override protected void onStart() { super.onStart(); if (getWeather == null) { getWeather = new GetWeather(); getWeather.execute(); } } public class GetWeather extends AsyncTask<Void,Void,List<Object>> { //süren işlemi göster private ProgressDialog loading; //json veri tiplerini belirle private JSONObject jsonReader ; private JSONObject main ; private JSONArray weather; private JSONObject sys; //json istenen verileri array at private List<Object> weatherList; //konum (GPS, NETWORK) servisi private GPSTracker gpsTracker; @Override protected void onPreExecute() { super.onPreExecute(); gpsTracker = new GPSTracker(MainActivity.this); weatherList = new ArrayList<Object>(); loading = ProgressDialog.show(MainActivity.this,"Please Wait...",null,true,true); } @Override protected List<Object> doInBackground(Void... params) { //tamponlu okuyucu BufferedReader bufferedReader = null; double latitude = 0; double longitude = 0; //konum bilgisi alınabiliyor mu? if(gpsTracker.canGetLocation()) { //enlem boylam bilgisini çek latitude = gpsTracker.getLatitude(); longitude = gpsTracker.getLongitude(); try { //json servisi için hedef url URL url = new URL("http://api.openweathermap.org/data/2.5/weather?lat="+latitude+"&lon="+longitude+"&units=metric"); //URL url = new URL("http://api.openweathermap.org/data/2.5/weather?q=Antalya&units=metric"); //java .net sınıfına ait http sağlayıcı HttpURLConnection con = (HttpURLConnection) url.openConnection(); StringBuilder sb = new StringBuilder(); bufferedReader = new BufferedReader(new InputStreamReader(con.getInputStream())); String json; while((json = bufferedReader.readLine())!= null){ sb.append(json+"\n"); } //okunan veriyi al jsonReader = new JSONObject(sb.toString().trim()); //verideki main anahtarı bilgilerini tut ve temp değerini listeye ekle main = jsonReader.getJSONObject(MAIN); weatherList.add(main.getString(TEMP)); //weather anahtarının gösterdiği diziyi al weather = jsonReader.optJSONArray("weather"); //dizinin ilk elemanını çek JSONObject jsonObject = weather.getJSONObject(0); //hava durumunu resim olarak göstermek için weather.icon bilgisini çek ve url' ye ata //url' ye ulaş ve bitmap olarak listeye ekle URL url1 = new URL("http://openweathermap.org/img/w/"+jsonObject.getString("icon")+".png"); Bitmap bmp = BitmapFactory.decodeStream(url1.openConnection().getInputStream()); weatherList.add(bmp); //aynı şekilde ülke kodunu çekmek için sys = jsonReader.getJSONObject(SYS); weatherList.add(sys.getString(COUNTRY)); //get(2) //şehir adını çekmek için weatherList.add(jsonReader.getString(CITY)); // GET(3) // diziyi onPostExecute metoduna gönder return weatherList; }catch (IOException e) { e.printStackTrace(); return null; } catch (JSONException e) { e.printStackTrace(); return null; } }else{ /* can't get location GPS or Network is not enabled Ask user to enable GPS/ network in settings alınmıyorsa onPostExecute null döndür */ return null; } } @Override protected void onPostExecute(List<Object> s) { super.onPostExecute(s); loading.dismiss(); //konum bilgisi alınamadıysa android setting activity' sine gönder if (s == null) { getWeather = null; gpsTracker.showSettingsAlert(); }else { //konum bilgisi alındıysa dizideki verileri context' e bas myTextView.setText((CharSequence) s.get(0).toString() + " \u00b0"); // ° Derece sembolü myTextViewCountry.setText((CharSequence) s.get(2)); getMyTextViewCity.setText((CharSequence) s.get(3)); myImageView.setImageBitmap((Bitmap) s.get(1)); //konum bilgisini toast a basmak isterseniz if (gpsTracker.canGetLocation()) { double latitude = gpsTracker.getLatitude(); double longitude = gpsTracker.getLongitude(); Toast.makeText(getApplicationContext(), "Your Location is - \nLat: " + latitude + "\nLong: " + longitude, Toast.LENGTH_LONG).show(); } else { gpsTracker.showSettingsAlert(); } //myWebView.loadUrl("http://openweathermap.org/img/w/03d.png"); } gpsTracker.stopUsingGPS(); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Adım 4 :
Konum Belirlemek İçin Gerekli Servisi Yazın.
package com.example.gurkan0791.weatherlikeapp; /** * Created by gurkan0791 on 05.10.2012. * bu bir copy-paste sınıftır. */ import android.app.AlertDialog; import android.app.Service; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.location.Location; import android.location.LocationListener; import android.location.LocationManager; import android.os.Bundle; import android.os.IBinder; import android.provider.Settings; import android.util.Log; public class GPSTracker extends Service implements LocationListener { private final Context mContext; // flag for GPS status boolean isGPSEnabled = false; // flag for network status boolean isNetworkEnabled = false; // flag for GPS status boolean canGetLocation = false; Location location; // location double latitude; // latitude double longitude; // longitude // The minimum distance to change Updates in meters private static final long MIN_DISTANCE_CHANGE_FOR_UPDATES = 10; // 10 meters // The minimum time between updates in milliseconds private static final long MIN_TIME_BW_UPDATES = 1000 * 60 * 1; // 1 minute // Declaring a Location Manager protected LocationManager locationManager; public GPSTracker(Context context) { this.mContext = context; getLocation(); } public Location getLocation() { try { locationManager = (LocationManager) mContext .getSystemService(LOCATION_SERVICE); // getting GPS status isGPSEnabled = locationManager .isProviderEnabled(LocationManager.GPS_PROVIDER); // getting network status isNetworkEnabled = locationManager .isProviderEnabled(LocationManager.NETWORK_PROVIDER); if (!isGPSEnabled && !isNetworkEnabled) { // no network provider is enabled } else { //ilk önce network metoduyla konum alıyor. //ama GPS açıksa network yerine gps yükleniyor. this.canGetLocation = true; // First get location from Network Provider if (isNetworkEnabled) { locationManager.requestLocationUpdates( LocationManager.NETWORK_PROVIDER, MIN_TIME_BW_UPDATES, MIN_DISTANCE_CHANGE_FOR_UPDATES, this); Log.d("Network", "Network"); if (locationManager != null) { location = locationManager .getLastKnownLocation(LocationManager.NETWORK_PROVIDER); if (location != null) { latitude = location.getLatitude(); longitude = location.getLongitude(); } } } // if GPS Enabled get lat/long using GPS Services if (isGPSEnabled) { if (location == null) { locationManager.requestLocationUpdates( LocationManager.GPS_PROVIDER, MIN_TIME_BW_UPDATES, MIN_DISTANCE_CHANGE_FOR_UPDATES, this); Log.d("GPS Enabled", "GPS Enabled"); if (locationManager != null) { location = locationManager .getLastKnownLocation(LocationManager.GPS_PROVIDER); if (location != null) { latitude = location.getLatitude(); longitude = location.getLongitude(); } } } } } } catch (Exception e) { e.printStackTrace(); } return location; } /** * Stop using GPS listener * Calling this function will stop using GPS in your app * */ public void stopUsingGPS(){ if(locationManager != null){ try { locationManager.removeUpdates(GPSTracker.this); }catch (SecurityException e) { e.printStackTrace(); } } } /** * Function to get latitude * */ public double getLatitude(){ if(location != null){ latitude = location.getLatitude(); } // return latitude return latitude; } /** * Function to get longitude * */ public double getLongitude(){ if(location != null){ longitude = location.getLongitude(); } // return longitude return longitude; } /** * Function to check GPS/wifi enabled * @return boolean * */ public boolean canGetLocation() { return this.canGetLocation; } /** * Function to show settings alert dialog * On pressing Settings button will lauch Settings Options * */ public void showSettingsAlert(){ AlertDialog.Builder alertDialog = new AlertDialog.Builder(mContext); // Setting Dialog Title alertDialog.setTitle("GPS is settings"); // Setting Dialog Message alertDialog.setMessage("GPS is not enabled. Do you want to go to settings menu?"); // On pressing Settings button alertDialog.setPositiveButton("Settings", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog,int which) { Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS); mContext.startActivity(intent); } }); // on pressing cancel button alertDialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { dialog.cancel(); } }); // Showing Alert Message alertDialog.show(); } @Override public void onLocationChanged(Location location) { } @Override public void onProviderDisabled(String provider) { } @Override public void onProviderEnabled(String provider) { } @Override public void onStatusChanged(String provider, int status, Bundle extras) { } @Override public IBinder onBind(Intent arg0) { return null; } }
Hiç yorum yok:
Yorum Gönder