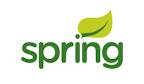
Apache Tiles kullanarak template nasıl hazırlanır ve kullanılır.
Başla
Apache Tiles Nedir?
bkz. Apache
bkz. Google
Kullanılan Teknolojiler
- Spring MVC 4
- Apache Tiles 3.0.5
- Netbeans IDE 8.0.2
Adım 1 :
Proje yapısını aşağıdaki gibi oluşturalım.
Adım 2 :
Apache Tiles için gerekli dependency ekleyelim (pom.xml)
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mycompany</groupId> <artifactId>MavenSpring</artifactId> <version>1.0-SNAPSHOT</version> <packaging>war</packaging> <name>MavenSpring</name> <properties> <endorsed.dir>${project.build.directory}/endorsed</endorsed.dir> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <jstl.version>1.2</jstl.version> <javax.servlet.version>3.0.1</javax.servlet.version> </properties> <dependencies> <dependency> <groupId>javax</groupId> <artifactId>javaee-web-api</artifactId> <version>7.0</version> <scope>provided</scope> </dependency> <!-- spring-context which provides core functionality --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.1.6.RELEASE</version> </dependency> <!-- The spring-aop module provides an AOP Alliance-compliant aspect-oriented programming implementation allowing you to define --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>4.1.6.RELEASE</version> </dependency> <!-- The spring-webmvc module (also known as the Web-Servlet module) contains Spring’s model-view-controller (MVC) and REST Web Services implementation for web applications --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>4.1.6.RELEASE</version> </dependency> <!-- The spring-web module provides basic web-oriented integration features such as multipart file upload functionality and the initialization of the IoC container using Servlet listeners and a web-oriented application context --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>4.1.6.RELEASE</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>${javax.servlet.version}</version> <scope>provided</scope> </dependency> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>${jstl.version}</version> </dependency> <!--Apache Tiles--> <dependency> <groupId>org.apache.tiles</groupId> <artifactId>tiles-extras</artifactId> <version>3.0.5</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>1.7</source> <target>1.7</target> <compilerArguments> <endorseddirs>${endorsed.dir}</endorseddirs> </compilerArguments> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>2.3</version> <configuration> <failOnMissingWebXml>false</failOnMissingWebXml> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-dependency-plugin</artifactId> <version>2.6</version> <executions> <execution> <phase>validate</phase> <goals> <goal>copy</goal> </goals> <configuration> <outputDirectory>${endorsed.dir}</outputDirectory> <silent>true</silent> <artifactItems> <artifactItem> <groupId>javax</groupId> <artifactId>javaee-endorsed-api</artifactId> <version>7.0</version> <type>jar</type> </artifactItem> </artifactItems> </configuration> </execution> </executions> </plugin> </plugins> </build> </project>
Adım 3 :
tiles.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 3.0//EN" "http://tiles.apache.org/dtds/tiles-config_3_0.dtd"> <tiles-definitions> <definition name="defaultTemplate" template="/WEB-INF/jsp/template/template.jsp"> <put-attribute name="header" value="/WEB-INF/jsp/template/header.jsp" /> <put-attribute name="menu" value="/WEB-INF/jsp/template/menu.jsp" /> <put-attribute name="body" value="/WEB-INF/jsp/template/body.jsp" /> <put-attribute name="footer" value="/WEB-INF/jsp/template/footer.jsp" /> </definition> </tiles-definitions>
Adım 4 :
Config.java
package com.mycompany.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.config.annotation.EnableWebMvc; import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter; import org.springframework.web.servlet.view.JstlView; import org.springframework.web.servlet.view.UrlBasedViewResolver; import org.springframework.web.servlet.view.tiles3.TilesConfigurer; import org.springframework.web.servlet.view.tiles3.TilesView; /** * * @author gurkan */ @Configuration @ComponentScan("com.mycompany") @EnableWebMvc public class Config extends WebMvcConfigurerAdapter{ @Bean public UrlBasedViewResolver viewResolver(){ UrlBasedViewResolver resolver = new UrlBasedViewResolver(); resolver.setPrefix("/WEB-INF/jsp/view/"); resolver.setSuffix(".jsp"); resolver.setViewClass(JstlView.class); resolver.setOrder(0); return resolver; } @Bean public UrlBasedViewResolver xmlViewResolver(){ UrlBasedViewResolver resolver = new UrlBasedViewResolver(); resolver.setViewClass(TilesView.class); resolver.setOrder(1); return resolver; } @Bean public TilesConfigurer tilesConfigurer() { TilesConfigurer tilesConfigurer = new TilesConfigurer(); tilesConfigurer.setDefinitions(new String[] { "/WEB-INF/tiles.xml" }); tilesConfigurer.setCheckRefresh(true); return tilesConfigurer; } }
Adım 5 :
WebInitializer.java
package com.mycompany.config; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.ServletRegistration.Dynamic; import org.springframework.web.WebApplicationInitializer; import org.springframework.web.context.support.AnnotationConfigWebApplicationContext; import org.springframework.web.servlet.DispatcherServlet; /** * * @author gurkan */ public class WebInitializer implements WebApplicationInitializer{ @Override public void onStartup(ServletContext sc) throws ServletException { AnnotationConfigWebApplicationContext ctx = new AnnotationConfigWebApplicationContext(); ctx.register(Config.class); ctx.setServletContext(sc); Dynamic servlet = sc.addServlet("dispatcher", new DispatcherServlet(ctx)); servlet.addMapping("/"); } }
Adım 6 :
HelloController.java
package com.mycompany.controllers; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; /** * * @author gurkan */ @Controller public class HelloController { @RequestMapping(value = "/" , method = RequestMethod.GET) public String index(ModelMap map) { map.put("hello", "Hello Spring MVC with Tiles"); return "index"; } @RequestMapping(value = "/page1", method = RequestMethod.GET) public String page1() { return "page1"; } @RequestMapping(value = "/page2", method = RequestMethod.GET) public String page2(ModelMap model) { return "page2"; } }
Adım 7 :
template.jsp
<%-- Document : template Author : gurkan --%> <%@page contentType="text/html" pageEncoding="UTF-8"%> <%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <c:set var="cp" value="${pageContext.request.servletContext.contextPath}" scope="request"></c:set> <!DOCTYPE html> <html> <head> </head> <body> <div > <div style="background-color: burlywood;"> <div > <tiles:insertAttribute name="header" /> </div> </div> <div > <div style="background-color: coral;"> <tiles:insertAttribute name="menu" /> </div> <div style="background-color: antiquewhite;"> <tiles:insertAttribute name="body" /> </div> </div> <div style="background-color: chartreuse;"> <div > <tiles:insertAttribute name="footer" /> </div> </div> </div> </body> </html>
Adım 8 :
body.jsp
<%-- Document : body Author : gurkan --%> <%@page contentType="text/html" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>JSP Page</title> </head> <body> <h1>Default Body</h1> </body> </html>
menu.jsp
<%-- Document : menu Author : gurkan --%> <%@page contentType="text/html" pageEncoding="UTF-8"%> <c:set var="cp" value="${pageContext.request.servletContext.contextPath}" scope="request"></c:set> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>JSP Page</title> </head> <body> <h1>List</h1> <ul class="nav nav-pills nav-stacked"> <li role="presentation" class="active"><a href="${cp}/">AnaSayfa</a></li> <li role="presentation"><a href="${cp}/page1">Page1</a></li> <li role="presentation"><a href="${cp}/page2">Page2</a></li> </ul> </body> </html>
Not: footer, header gibi elementleri çoğaltabilirsiniz.
Adım 9 :
Oluşturulan template' i kullanmak için
index.jsp
<%-- Document : index Author : gurkan --%> <%@page contentType="text/html" pageEncoding="UTF-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%> <c:set var="cp" value="${pageContext.request.servletContext.contextPath}" scope="request"></c:set> <!DOCTYPE html> <tiles:insertDefinition name="defaultTemplate" > <tiles:putAttribute name="body" > <h1>Hello Body!</h1> <h2>${hello}</h2> </tiles:putAttribute> </tiles:insertDefinition>
Adım 10 :
Projeyi Build ve Run edelim.
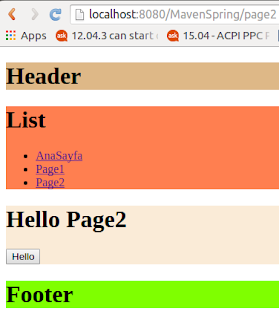
b ) page1.jsp Ekran Görüntüsü
c ) page2.jsp Ekran Görüntüsü
Spring MVC 4 Maven + AngularJS + Bootstrap Entegrasyonu İçin Buradan Geçebilirsiniz.
Hiç yorum yok:
Yorum Gönder